The matlab code is about images and i havent been able to figure out to solve my problem
5 views (last 30 days)
Show older comments
Project Overview
In this project we will be implementing a series of image alterations. We will create a function that calls other helper functions based on type of alteration chosen. The original and altered image are both displayed in two separate figures.
Part 1: The Main Function - convertImage
The convertImage function should output an altered image array and take in two parameters. The first parameter is an image array that is the starting point for the alteration, and the second parameter is a numerical value (1, 2, or 3) that indicates which alteration helper function to call.
Alteration Value Possibilities
- 1 corresponds to the blurring image alteration
- 2 corresponds to the distorting image alteration
- 3 corresponds to the grayscale image alteration.
- When the second parameter is not one of these values the function should simply output the starting point array.
This function should display the images.
HINT: While vectorization may be possible for portions of a helper function, all three of the helper functions modify individual pixels and therefore similar code can be in each to iterate over the image array.
Part 2: Helper Function - Blurring the Image
This helper function should output the altered image array and take in a single parameter that contains an image array to use as the starting point for the alteration.
Start this function by determining the size of the image array, and setting up your output variable to be equivalent to the starting point image array. Test out your code at this point. Your program should now produce a successful execution that goes through the entire script area and can call this helper function but the script area's display of the original and altered images should look the same since no changes have been made to the array.
To blur the image, you will alter all the interior pixels of the image. Each pixel should be updated to a value equivalent to its local mean. To calculate the local mean, we can take the mean of all the neighbors of the current pixel in addition to itself (9 pixels).
Recall that a neighbor is the adjacent or surrounding pixels or values in an array. For example in the following 3x4 array the second row and third column contains the value 7 and it has 8 neighbors (1, 6, 10, 5, 12, 9, 2, and 15) which all together create a local mean of (1+6+10+5+7+12+9+2+15) / 9.
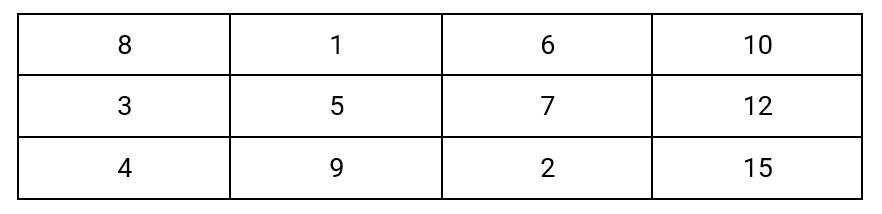
This function should not display anything to the command window nor should it display the images.
Part 3: Helper Function - Distorting the Image
This helper function should output the altered image array and take in a single parameter that contains an image array to use as the starting point for the alteration.
Start this function by determining the size of the image array, and setting up your output variable to be equivalent to the starting point image array. Test out your code at this point. Your program should now produce a successful execution that goes through the entire script area and can call this helper function but the script area's display of the original and altered images should look the same since no changes have been made to the array.
To distort the image we will move the quadrants of the image around. Here is a reference to the quadrant numbers we will use, where 1 is the top-left corner of the image:
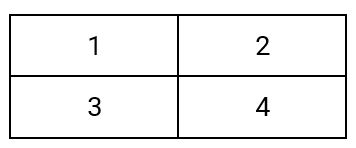
You may assume the image will always have an even number of rows and columns.
HINT: You may want to determine the halfway value that separates the quadrants to help with your coding.
To complete our distortion we will update our output array to change the values in each quadrant. The first quadrant's values will be updated to be values of the fourth quadrant in the original image array. The second quadrant's values will be updated to be the values of the first quadrant in the original image array. The third quadrant's values will be updated to be the values of the second quadrant in the original image array. Finally the fourth quadrant's values will be updated to be the values of the third quadrant in the original image array. Once complete, our reorganization will result in an image array that looks like this:
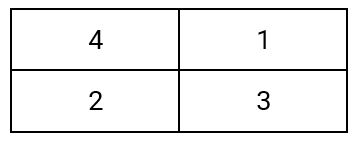
This function should not display anything to the command window nor should it display the images.
Part 4: Helper Function - Converting the Image to Grayscale
This helper function should output the altered image array and take in a single parameter that contains an image array to use as the starting point for the alteration.
Start this function by determining the size of the image array, and setting up your output variable to be equivalent to the starting point image array. Test out your code at this point. Your program should now produce a successful execution that goes through the entire script area and can call this helper function but the script area's display of the original and altered images should look the same since no changes have been made to the array.
For our grayscale conversion we will use the formula of 1.25 * mean(R, G, B) where R, G, and B are the individual color values for a specific pixel of the input image array. You must update each pixel's value in the output image array to be a new value based on that formula.
For example given a pixel value of [255, 50, 255] (a bright pink) then using the formula the pixel will be set to [1.25*(255+50+255)/3, 1.25*(255+50+255)/3, 1.25*(255+50+255)/3]. Here is the before and after of the single pixel:

Finally, once all the pixel by pixel alterations are complete we have one more task to do when the image size has a 3rd dimension (meaning it's in color). In this case, we need to eliminate the third dimension of the image as a grayscale image array only has two dimensions. One way to do this is to first delete the blue layer and then delete the green layer.
This function should not display anything to the command window nor should it display the images.
Submission
zyBooks Submission
Copy in your main convertImage function and any helper functions. The first line on zyBooks should be the start of the convertImage function definition. You must do this to verify your code works within zyBooks as a similar harness will be utilized by the graders when grading.
Style Grading
Style is the readability of your code. As we progress through the semester, style will become important to help reduce the amount of time debugging your code as poor style can quickly lead to syntax errors and code misinterpretation. For project 5 the following items will be checked:
- Your submission has a proper comment header.
- Your submission has visible white space allowing another person to easily read the code you have submitted. This whitespace could include but is not exclusive to blank lines between implementation steps, spacing around mathematical operations and assignments.
- Your code should contain useful human readable comments that help explain in your own words what code blocks (multiple lines of code) do.
- Your code does not produce excessive superfluous output. (remember your semicolons)
- Consistent and proper indentation within branches, loops, and functions
this is my code
function converted = convertImage(im, alterationType)
% Main function to alter the image based on alterationType
% Parameters:
% - im: the input image array
% - alterationType: numerical value (1, 2, or 3) indicating the type of alteration
% Display original image
figure;
subplot(1, 2, 1);
imshow(im);
title('Original Image');
% Call the appropriate helper function based on alterationType
switch alterationType
case 1
% Blurring image alteration
converted = blurImage(im);
title('Blurred Image');
case 2
% Distorting image alteration
converted = distortImage(im);
title('Distorted Image');
case 3
% Grayscale image alteration
converted = grayscaleImage(im);
title('Grayscale Image');
otherwise
% No alteration for unknown alterationType
converted = im;
title('No Alteration');
end
% Display altered image
subplot(1, 2, 2);
imshow(converted);
end
function blurred = blurImage(im)
% Helper function to blur the image
[rows, cols, ~] = size(im);
blurred = im;
for i = 2:rows-1
for j = 2:cols-1
% Calculate local mean for each pixel
localMean = mean(mean(im(i-1:i+1, j-1:j+1, :)));
blurred(i, j, :) = localMean;
end
end
end
function distorted = distortImage(im)
% Helper function to distort the image
[rows, cols, channels] = size(im);
halfway = cols / 2;
distorted = im;
% Swap quadrants to distort the image
if channels == 3
% For color images
distorted(1:rows/2, 1:halfway, :) = im(rows/2+1:end, halfway+1:end, :);
distorted(1:rows/2, halfway+1:end, :) = im(rows/2+1:end, 1:halfway, :);
distorted(rows/2+1:end, 1:halfway, :) = im(1:rows/2, halfway+1:end, :);
distorted(rows/2+1:end, halfway+1:end, :) = im(1:rows/2, 1:halfway, :);
else
% For grayscale images
topRight = im(1:rows/2, halfway+1:end);
bottomLeft = im(rows/2+1:end, 1:halfway);
distorted(1:rows/2, 1:halfway) = bottomLeft;
distorted(1:rows/2, halfway+1:end) = topRight;
distorted(rows/2+1:end, 1:halfway) = topRight;
distorted(rows/2+1:end, halfway+1:end) = bottomLeft;
end
end
function grayscale = grayscaleImage(im)
% Helper function to convert the image to grayscale
[rows, cols, channels] = size(im);
grayscale = im;
for i = 1:rows
for j = 1:cols
% Calculate grayscale value using the formula
grayscale(i, j, :) = 1.25 * mean(im(i, j, :));
end
end
% Eliminate the third dimension for color images
if channels == 3
grayscale = squeeze(grayscale(:, :, 1));
end
end
this is the problem
Alter Color Image: convertImage(im, 2) - Expected to distort the image.0% (11%)
Variable studentResult has an incorrect value.
Assessment result: incorrectAlter Grayscale Image: convertImage(im, 2) - Expected to distort the image.0% (11%)
Variable studentResult has an incorrect value.
Assessment result: incorrectDouble Call of convertImage(im, 2) - Expected to distort the image twice.0% (11%)
Variable studentResult has an incorrect value.
hen calling the required convertImage function with the option for distorting the image and then calling it again with the result, the output from convertImage was not an image array containing a properly distorted image.
1 Comment
Voss
on 20 Nov 2023
Notice that the second time you call title(), inside one of the case blocks, that title overwrites the first title because you haven't created the second subplot yet, and the second subplot remains untitled.
Answers (1)
Walter Roberson
on 20 Nov 2023
All four quadrants of the original image need to appear in the distorted image, but in the case of grayscale you coded
distorted(1:rows/2, 1:halfway) = bottomLeft;
distorted(1:rows/2, halfway+1:end) = topRight;
distorted(rows/2+1:end, 1:halfway) = topRight;
distorted(rows/2+1:end, halfway+1:end) = bottomLeft;
which outputs tthe bottomLeft and topRight twice and discards topLeft and bottomRight.
Hint: consider your existing code
distorted(1:rows/2, 1:halfway, :) = im(rows/2+1:end, halfway+1:end, :);
what would happen if you tried to execute that when im is a 2D array?
2 Comments
Walter Roberson
on 20 Nov 2023
See @Voss's Answer; Voss shows the code you need for 3D and explains why you do not need different code for 2D.
See Also
Categories
Find more on Image Processing Toolbox in Help Center and File Exchange
Community Treasure Hunt
Find the treasures in MATLAB Central and discover how the community can help you!
Start Hunting!